Engage audiences like never before
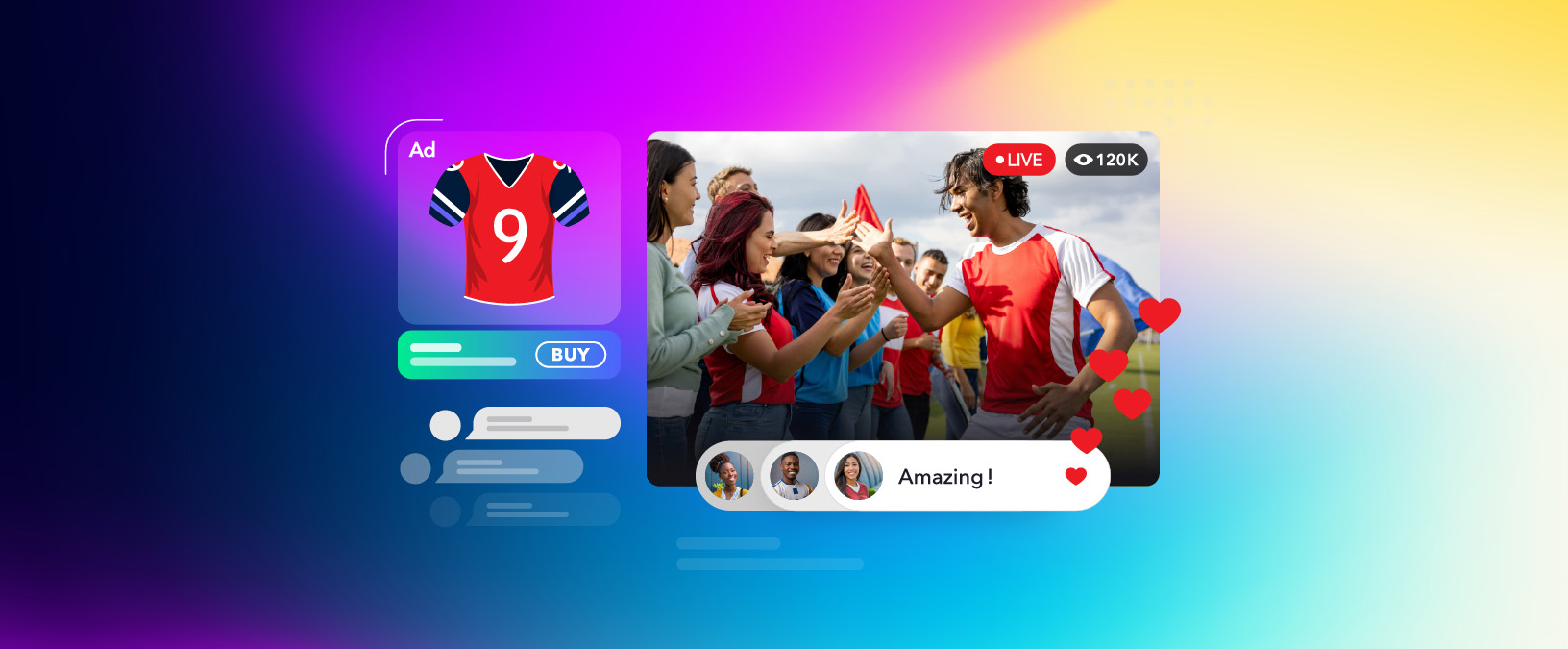
Transforming Fan Engagement in Sports: Going Beyond the Game
Sports fan engagement is rapidly evolving into a year-round, personalized, and immersive …
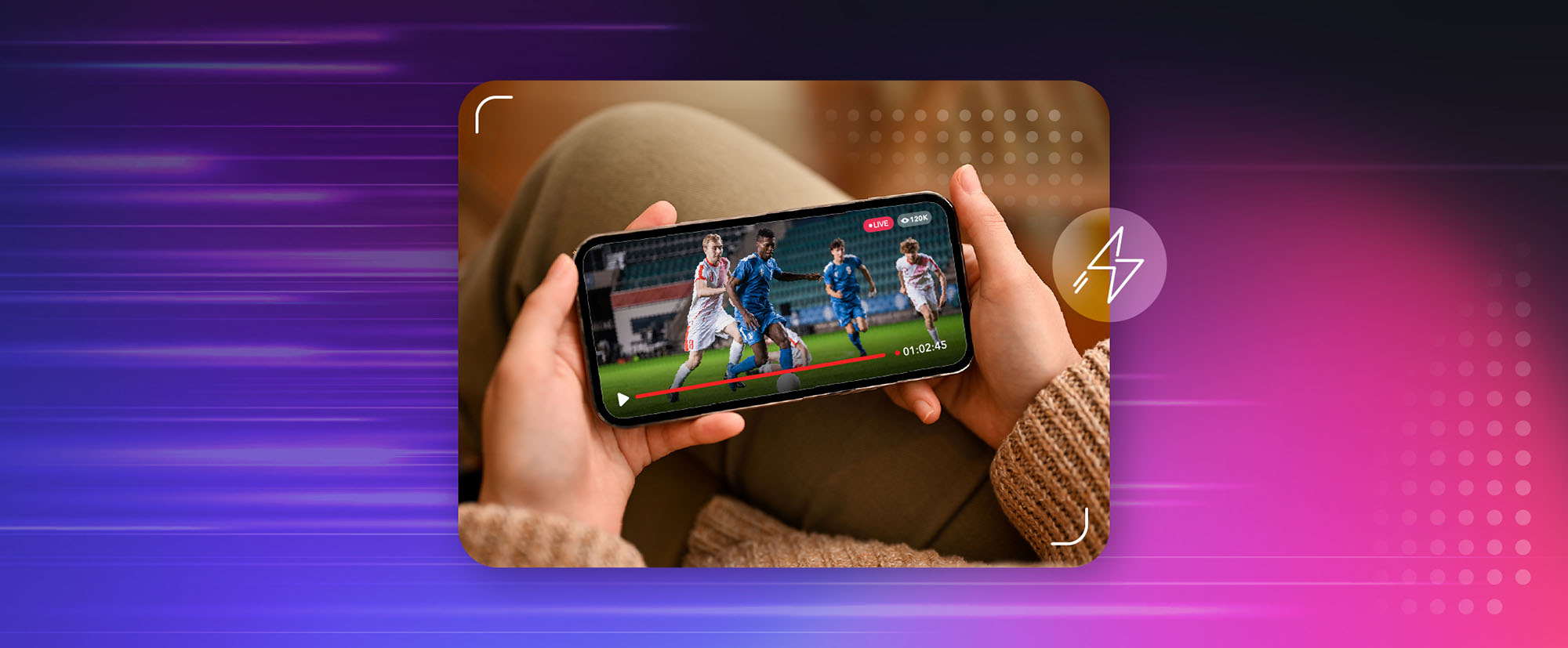
The Complete Guide to Low Latency in Live Streaming
Low latency has become a critical factor in live streaming across various …
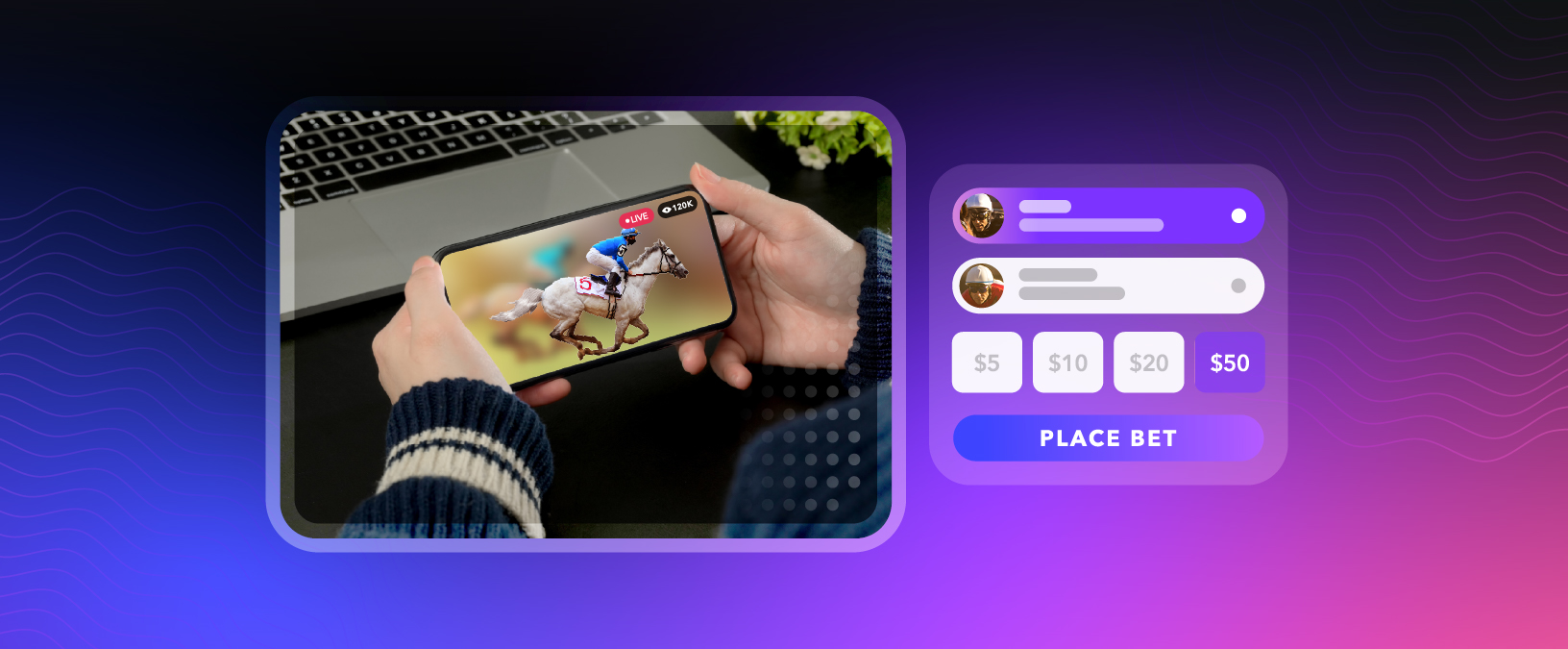
The Digital Rebirth of Live Horse Racing: How Technology is Fueling iGaming’s Rapid Rise
The digital transformation of horse racing happening, along with the iGaming revolution, …
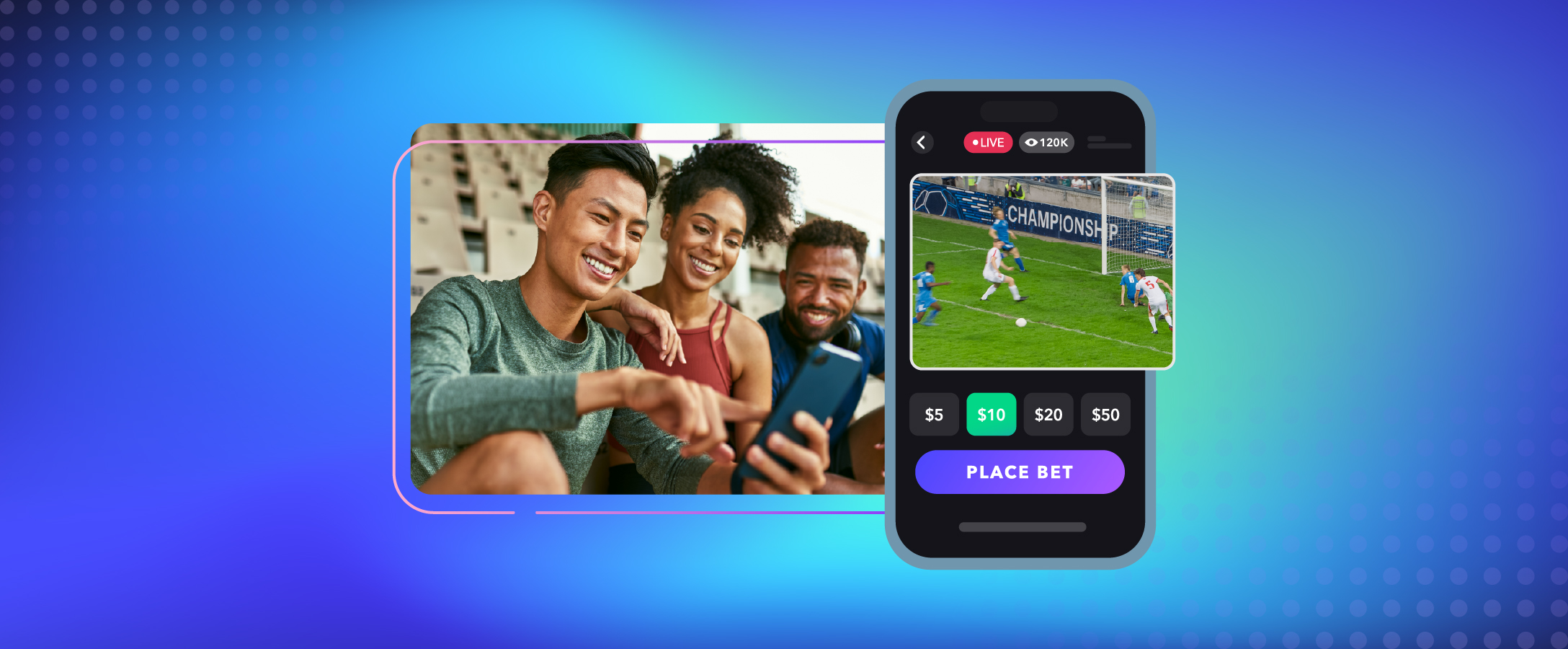
Boost Viewer Engagement: 5 Pro Tips for Your Live Sports Broadcast
The future of live sports lies in providing interactive, immersive, and personalized …